Basic Http requests
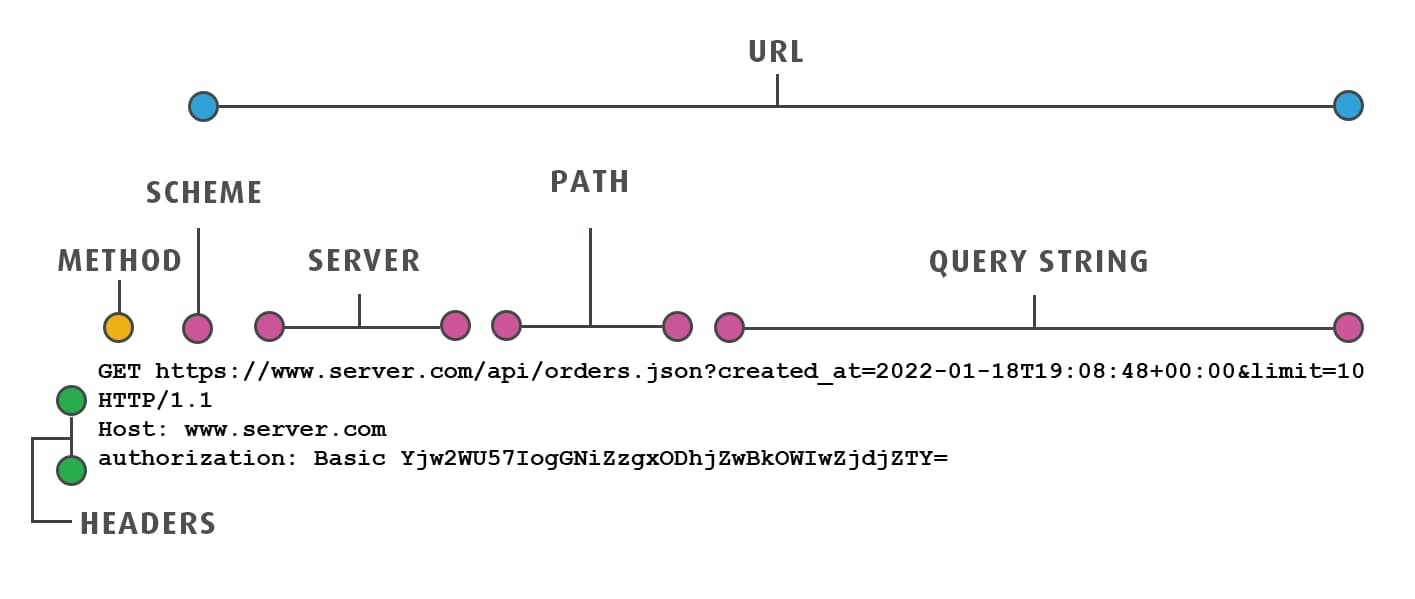
- GET /search?q=test HTTP/2
- Host: www.bing.com
- User-Agent: curl/7.54.0
- Accept: /
The host
Host: www.bing.com
In the above, the second line with ‘Host’, defines which website the request is to be made to. In this case, the request will be sent to the Bing website. If this was changed to ‘www.google.com’, the request would go to Google.
The method
GET /search?q=test HTTP/2
- GET — retrieves data, like a blog article.
- POST — creates data, like a new blog article.
- PUT — replaces data, like an existing blog article.
- DELETE — deletes data, like an existing blog article.
So, our example request using the GET method, says: ‘Please retrieve some data for me.’
There are other HTTP methods which can be seen in these MDN web docs.
The Path

GET /search?q=test HTTP/2
In the above, /search on the first line is the path. Coupled with the method, the path tells the website what the request specifically wants to do.
In this case, GET /search says: ‘Please retrieve the results of a search for me.‘
Headers
User-Agent: curl/7.54.0 Accept: * / *
In the above, the lines under the Host are headers. Headers are additional data that help a website determine what response to make for the request.
For example, Accept: / tells the website that any type of response is okay. This could be made more specific. User-Agent: curl/7.54.0 tells the website which application is used to make the request.
For example, this might typically say something like Mozilla/5.0 (Macintosh; Intel Mac OS X 10.14; rv:68.0) Gecko/20100101 Firefox/68.0 if you’re making the request from the Mac version of Firefox.
In the above case, a command-line tool, cURL, was used to make the request.
Any additional headers may be provided on following lines. A famous type of header is the ‘cookie’ header, which is used to pass reusable data to the website on each request.
For example, if logged-in, a user’s ‘session’ may be passed each time to the website so a user doesn’t have to pass a username and password each time a request is made.
Query String
GET /search ?q=test HTTP/2
In the above, q is an argument and test is a value. This is passed to the website’s server and provides additional information on how to fulfill the request.
In the case of our request specifically, we are now saying: ‘Please retrieve the results of a search for me, where the search value is ‘test’.’
If we need to pass additional values in the query string, we separate them with an ampersand (&) like so:
?q=test&query_argument_2=value_2&query_argument_3=value=3
Request body

A query string is not the only way to pass additional information to the website. A request body is a means of doing the same.
It is typically not used with a GET request but I’ve added it above for the sake of an example. Typically, it is used with methods such as POST and PUT which are heavier on data transmission as they specify data to be recorded.
In the above, after the headers and an empty line, ‘q’: ‘test’ is the request body which provides additional information to the website to help it fulfill its request, much like a query string does.
Passing data in the body has a few advantages to a query string and a few disadvantages.
Advantages
- There is no data size limit. Query strings have a maximum size limit (specific to the browser) and therefore aren’t good for transmitting a large amount of information.
- The data is recorded and retrievable in fewer places and is, therefore, more secure. Placing sensitive data, like passwords, in query strings is problematic (read more in this HttpWatch article).
- Better for sending other data transmission formats, such as JSON and XML. While these formats can be placed in a query string, it isn’t typically done. Query strings look extremely ugly with JSON or XML blobs in them.
Disadvantages
- The request body is hidden from the user and cannot be easily shared with another person. Query strings are good for ‘hey, check out this link’ situations since the values are stored in the link and can easily be copy/pasted to another person.
The HTTP version
GET /search?q=test HTTP/2
In the above, HTTP/2 on the first line is the HTTP version. Different versions of HTTP handle the details of the interaction between machines differently. The versions commonly used today are HTTP/1.1 and HTTP/2.
You can read more about different HTTP versions on MDN web docs.
How Http messages are sent

Just about all of the world’s HTTP communication is carried over TCP/IP, a popular layered set of packet-switched network protocols spoken by computers and network devices around the globe. A client application can open a TCP/IP connection to a server application, running just about anywhere in the world. Once the connection is established, messages exchanged between the client’s and server’s computers will never be lost, damaged, or received out of order.[1]
Say you want the latest power tools price list from Joe’s Hardware store:
